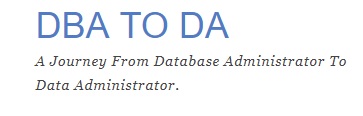
OEM 13cR3: Get List of All Targets (or Hosts)
In this short blog I am going to tell about on how to list all targets monitored by EM or filter specific targets
emcli list -resource=Targets -columns="TARGET_NAME:70,TARGET_TYPE:50"
And if you need to filter filter by type
emcli list -resource=Targets -columns="TARGET_NAME:70,TARGET_TYPE:50" | grep hosts